Vue Js Credit Card Expiry Date Validation:Vue.js can be used to implement credit card expiry date validation by leveraging its reactive data-binding and computed properties features. To perform validation, an input field can be bound to a Vue data property representing the expiry date. A computed property can be used to check if the entered date is valid by comparing it with the current date. If the expiry date is earlier than the current date, an error message can be displayed.
How can I implement credit card expiry date validation in Vue.js?
This code snippet is a Vue.js component that provides credit card expiry date validation. It consists of an input field for the expiry date, an error message display area, and a validation button.
The input field is bound to the expiryDate
data property using v-model
, which allows the user to enter the expiry date in the format MM/YY. The @input
event triggers the formatExpiryDate
method, which formats the entered date by removing non-digit characters and adding a slash (/) between the month and year.
The validateExpiryDate
method is called when the validation button is clicked. It checks if the input field is empty and displays the corresponding error message. If the field is not empty, it compares the entered date with the current date to determine if it is a valid expiry date. If it is valid, a success message is displayed; otherwise, an invalid expiry date error message is shown.
Overall, this code provides a basic client-side validation for credit card expiry dates using Vue.js.
Vue Js Credit Card Expiry Date Validation Example
<div id="app">
<input type="text" v-model="expiryDate" @input="formatExpiryDate" placeholder="Expiry Date (MM/YY)" :maxlength="5">
<div class="error-message" :class="{ 'success-message': showSuccess }"
v-if="showError || showSuccess || showEmptyError">
{{ showError ? errorMessage : (showEmptyError ? 'Input field required' : successMessage) }}
</div>
<button @click="validateExpiryDate">validate</button>
</div>
<script>
const app = Vue.createApp({
data() {
return {
expiryDate: '',
showError: false,
showSuccess: false,
errorMessage: '',
successMessage: '',
showEmptyError: false // New property
};
},
methods: {
formatExpiryDate() {
// Remove any non-digit characters
let formattedDate = this.expiryDate.replace(/\D/g, '');
// Add a slash (/) between month and year
if (formattedDate.length > 2) {
formattedDate = formattedDate.slice(0, 2) + '/' + formattedDate.slice(2);
}
this.expiryDate = formattedDate;
},
validateExpiryDate() {
const currentYear = new Date().getFullYear() % 100;
const currentMonth = new Date().getMonth() + 1;
if (this.expiryDate === '') {
// Input field is empty, display the empty error message
this.showError = false;
this.showSuccess = false;
this.showEmptyError = true;
this.errorMessage = 'Input field required';
} else {
const enteredYear = parseInt(this.expiryDate.slice(3), 10);
const enteredMonth = parseInt(this.expiryDate.slice(0, 2), 10);
if (
enteredYear < currentYear ||
(enteredYear === currentYear && enteredMonth < currentMonth) ||
enteredMonth < 1 ||
enteredMonth > 12
) {
this.showError = true;
this.showSuccess = false;
this.showEmptyError = false;
this.errorMessage = 'Invalid expiry date';
} else {
this.showError = false;
this.showSuccess = true;
this.showEmptyError = false;
this.successMessage = 'Valid expiry date';
}
}
}
}
});
app.mount('#app');
</script>
Output of Vue Js Credit Card Expiry Date Validation
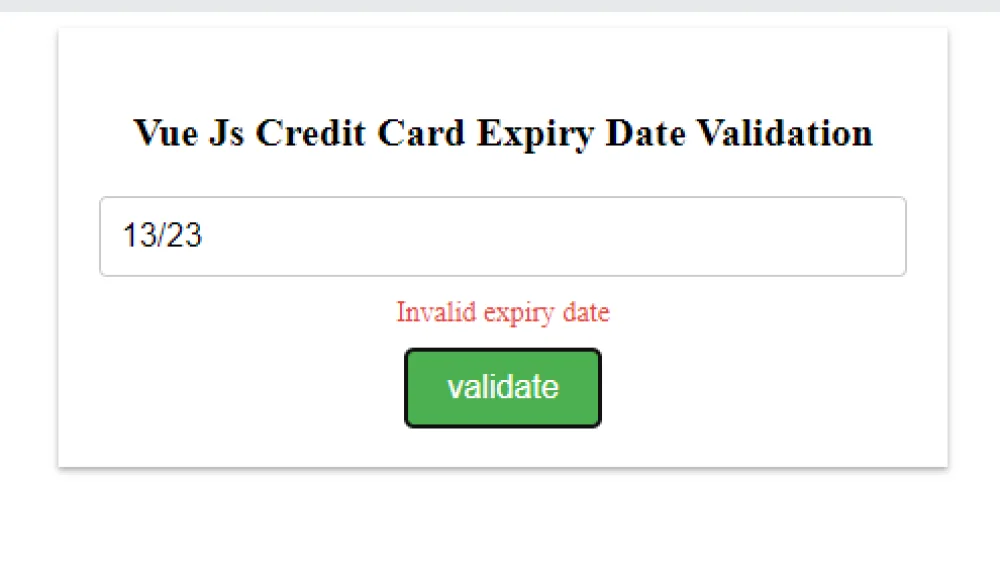